skip to “the new way” for how to do so
as it turns out… on ubuntu 22.10 and onward stops using the config file for ports…
so the old way was
vi /etc/ssh/sshd_config
and literally one line in there, “Port 2077”
(I also do PubkeyAuthentication yes, PasswordAuthentication no, UsePAM yes — I think the first and third settings are actually the default settings, so only need to disable password and add in port).
sudo service ssh restart
and just append your pubkeys to ~/.ssh/authorized_keys
so on reboot switched back to old port. I was using say 2077.
netstat | grep 2077
no services listed.
I got clued into this using sudo service ssh status and it said
$ sudo service ssh status
Oct 31 15:58:54 sffpc systemd[1]: Starting OpenBSD Secure Shell server…
Oct 31 15:58:54 sffpc sshd[6683]: Server listening on :: port 22.
And I went back to /etc/ssh/sshd_config and saw the lines
14 # Port and ListenAddress options are not used when sshd is socket-activated,
15 # which is now the default in Ubuntu. See sshd_config(5) and
16 # /usr/share/doc/openssh-server/README.Debian.gz for details.
you can also read more at
the docs
vi /usr/share/doc/openssh-server/README.Debian.gz
or https://github.com/namedun/debian-openssh/tree/master/debian
https://discourse.ubuntu.com/t/sshd-now-uses-socket-based-activation-ubuntu-22-10-and-later/30189
The provided ssh.socket unit file sets ListenStream=22. If you need to have it listen on a different address or port, then you will need to do this as follows (modifying ListenStream to match your requirements): mkdir -p /etc/systemd/system/ssh.socket.d cat >/etc/systemd/system/ssh.socket.d/listen.conf <<EOF [Socket] ListenStream=2222 EOF systemctl daemon-reload See systemd.socket(5) for details.
tl;dr the new way

Okay, so what I actually did was just edit the file with vi (you can use nano) since I was having issues with bash balking at creating the file etc.
$ sudo mkdir -p /etc/systemd/system/ssh.socket.d $ sudo vi /etc/systemd/system/ssh.socket.d/listen.conf $ sudo service ssh reload
And now I can ssh from another computer yay!
notes
I think in the future I will just use the default port. too much headache.
ufw
131 sudo ufw status
132 sudo ufw enable
130 sudo ufw allow ssh
160 sudo ufw default deny incoming
746 sudo ufw default allow outgoing
174 sudo ufw allow 2077
179 sudo ufw delete allow 22
180 sudo ufw status
181 sudo ufw delete allow 22/tcp
749 sudo ufw reload
logs
journaltcl -u ssh
router
also have to set up port forwarding on router.
https://www.asus.com/us/support/FAQ/114093/
WAN -> virtual server /port forwarding. Add profile: Protocol TCP, external port 20whatever, internal port 2077, Internal IP (find your computer on the list of stuff connected to router)
https://www.asus.com/us/support/FAQ/114093/
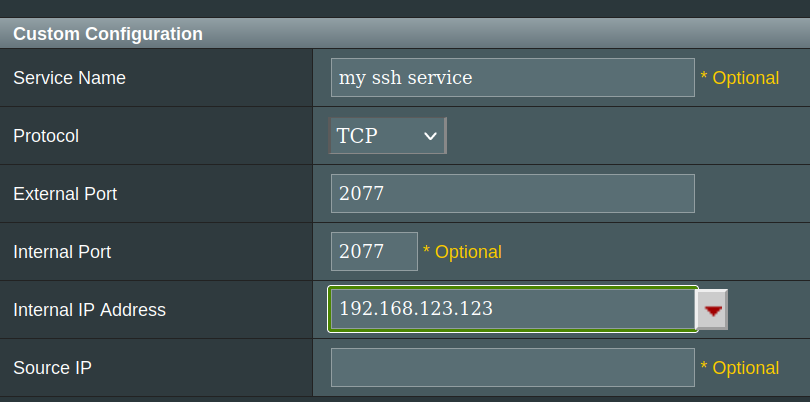
get external ip
curl ifconfig.me
side note
on mac was getting
debug1: Authenticator provider $SSH_SK_PROVIDER did not resolve; disabling
this was red herring; if it gets to “connection refused” that means we were able to make contact with the server, and it’s something on the server side.