Recently I had “great fun” modifying bootstrap v3’s image gallery slideshow thingy, Carousel (click here to see an interactive example).
It has some nice features built-in, like indicators for what slide you are on, auto-scaling so it works on any browser window size, and an easy way to select options like cycling the carousel continuously, changing the interval between auto-slides, or reacting to keyboard events.
I made some modifications to get it to look and behave the way I wanted. Namely, I wanted readable captions underneath the images. Bootstrap has the captions overlaid on the image by default, and white-on-white doesn’t work so well.
I mostly succeeded… here’s a screenshot:
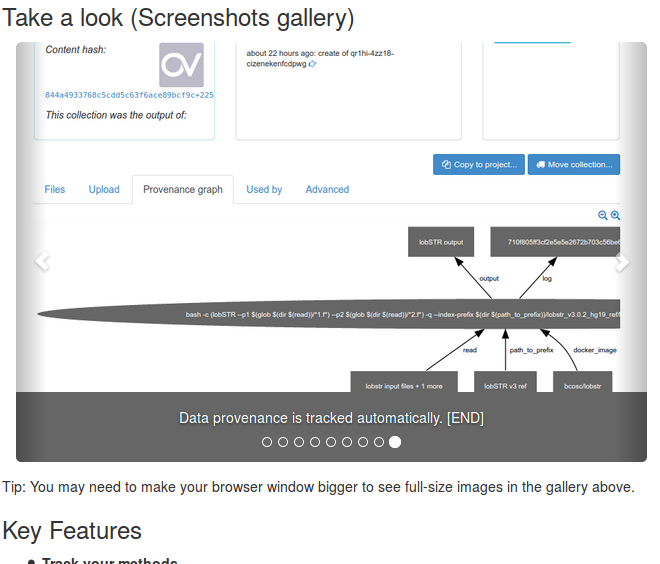
…although I still dislike how verbose the Carousel code is. Oh well, our docs site was already using Bootstrap.
The how-to on bootstrap’s page is pretty terse and kind of confusing for me, so I’m writing up a more detailed explanation here.
stuff needed
From http://getbootstrap.com/getting-started/, download the zip file. You’ll need
1. css/bootstrap.css (you can use the .min version if you’d like, which is just compressed into non-human-readable form)
2. js/bootstrap.js (you can also get away with just carousel.js).
You’ll also need jquery.js from http://jquery.com/download/, try the link “Download the compressed, production jQuery 1.11.2”.
Note: We’ll include the javascript files at the end of HTML so most of the page loads quicker.
how to
Alright, now how do you include all these files? (see this gist for the full HTML file, or download a self-contained zip file of all the files: bootstrap-carousel-example).
Put the CSS at the top, either by linking to a .css file or including the CSS directly:
<head> <link href="./css/bootstrap.css" rel="stylesheet"> <style> /* put your custom CSS here if you'd like, for instance... */ .carousel { margin: 1em; } </style>
Inside the body section, put your Carousel HTML. It’s kind of long so just check out the gist. Put the javascript at the bottom, either by linking to a .js file or else including it directly
<script src="./js/jquery.min.js"></script> <script src="./js/bootstrap.min.js"></script> <script type="text/javascript"> // Put your custom javascript options here, for instance... $(document).ready(function() { $('.carousel').carousel({interval: 100}); }); </script> </body> </html>
step 3: profit?
If you load the html file in your browser, magic should now happen.
For reference, you can download this zip file where everything is set-up already: bootstrap-carousel-example
step 4: customize
What if we want the images to cycle faster, or the images to not cycle at all?
Okay, I’ve already shown how you can select some of the built-in bootstrap options using javascript:
$(document).ready(function() { $('.carousel').carousel({interval: 100}); });
You can select individual carousels on a page by naming the carousel
<div id="carousel-keyfeatures" class="carousel slide" data-interval="false">
and then using a more specific jquery call
$(document).ready(function() { $('.carousel-keyfeatures').carousel({interval: 100}); });
Alternatively, if you want some option to apply to all carousels on a page / site, you can pull this code out into a separate file
./js/carousel-override.js
and include it in each HTML file
<script src="./js/carousel-override.js"></script>
A third way to change an the options on a carousel is to include it in the HTML, like so:
<div id="carousel-example-generic" class="carousel slide" data-ride="carousel" data-interval="false">
step 5: sweeter customizations!
Now, to make more custom modifications, I had to fiddle with the CSS. A few hours later, I made the modifications you can see at this gist. Alternatively, download the zip file and uncomment the line
<link href="./css/carousel-override.css" rel="stylesheet">
trouble-shooting tips (bugs!)
First, cool tools:
1) Use firefox or chrome’s powerful built-in developer tools. The default shortcut to bring them up is ctrl-shift-c (you actually can’t get rid of our change this shortcut easily, which is annoying).
You can delete things, edit things without refreshing the page or modifying files, and other wonderful tools.
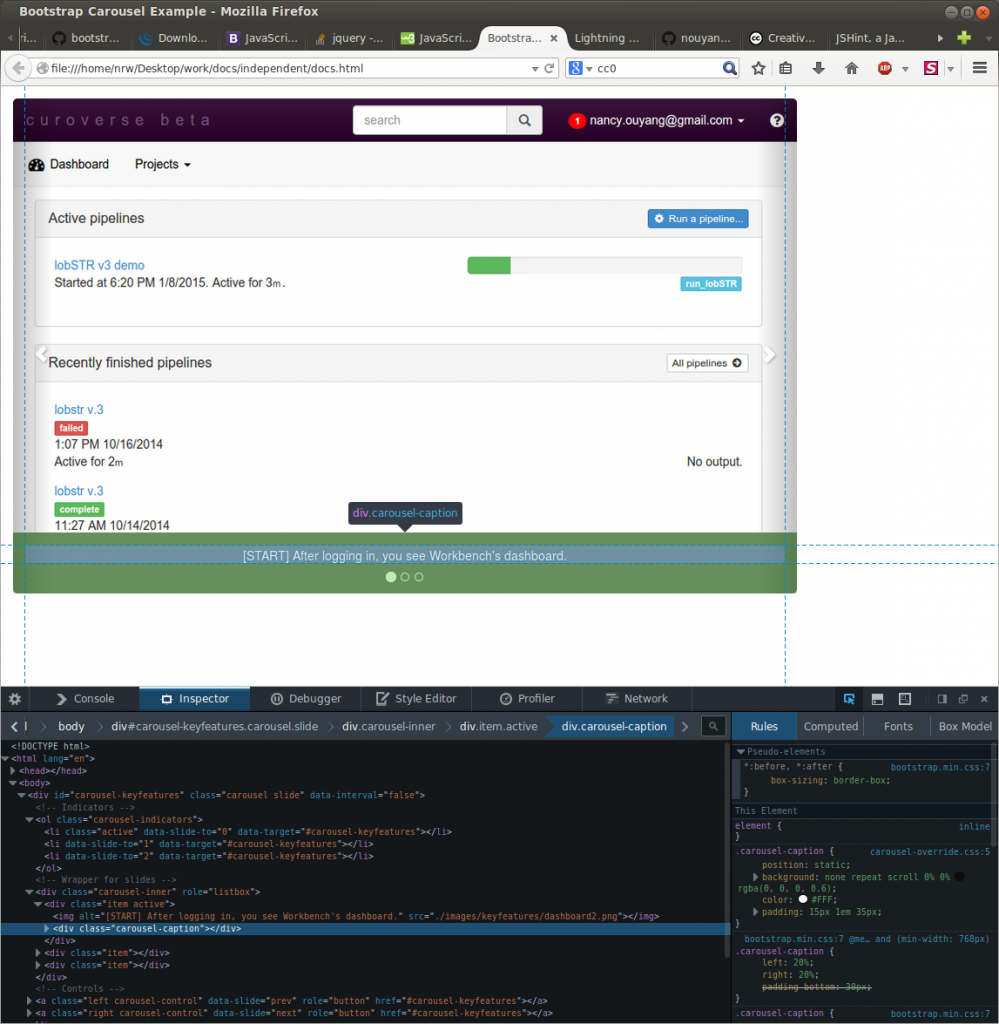
2) Use a Javascript hint tool, such as http://jshint.com/.
If you just use the Console tool in Firefox Inspector to see the javascript error messages, it is fairly cryptic.
SyntaxError: missing ) after argument list
Tools like JSHint can provide some more helpful feedback about what went wrong.
bugs
these things tripped me up:
1) the Bootstrap website makes ( and { look very similar on my computer, so I skipped the curly brackets and used
$('.carousel').carousel( interval: 2000 )
instead of the correct
$('.carousel').carousel({ interval: 2000 })
UPDATE 03 April 2015
I filed a bug on github: https://github.com/twbs/bootstrap/issues/16225, or as follows:
Font in code blocks on http://getbootstrap.com/ does not distinguish { and ( clearly, can cause anguish for newcomers to javascript. Please remove the Courier New
font fallback option. Just monospace
works well.
In Detail
I was following the the example at http://getbootstrap.com/javascript/#carousel, and my brain removed the “extra parenthesis” I think, similar to how you don’t notice I doubled “the” in this sentence unless I point it out.
This is a screenshot of the bootstrap website “code” block font currently on my computer:
Please remove the courier new
font fallback option. Just monospace
works well.
code, kbd, pre, samp {
font-family: monospace;
}
Results in the much-better
(Most open-source projects in have nice websites but no issue tracker nor contact info for their own website or their documentation, only for their github repo around the actual code, and it’s not always clear where to file a bug…)
END UPDATE
2) I didn’t realize I needed to include the jquery file first, so I got cryptic messages like “$ is undefined”.
3) I spent a while debugging a weird gap that left the rightmost controls in midair. It occurred when the browser window was too big. Turns out I needed to hardcode a max-width, which I just hardcoded to be slightly smaller than the smallest image width I had.
appendix
Here are the files used in this tutorial again: